How to fix “SyntaxError: Missing parentheses in call to ‘print'” in Python
The Python error “SyntaxError: Missing parentheses in call to ‘print’ …” occurs when you use an old-style print statement (e.g., print 'some value'
) in Python 3.
This not so short error message looks like this:
File /dwd/sandbox/test.py, line 1
print 'some text here'
^^^^^^^^^^^^^^^^^^^^^^
SyntaxError: Missing parentheses in call to 'print'. Did you mean print(...)?
As the error explains, from version 3, print()
is a function in Python and must be used as an ordinary function call:
# 🚫 This style of print statements only work in older versions of Python
print 'hello world'
# ✅ To fix the issue, we add parentheses
print('some text here')
You might get this error if you're running an old code in Python 3 or have copied a code snippet from an old post on Stackoverflow.
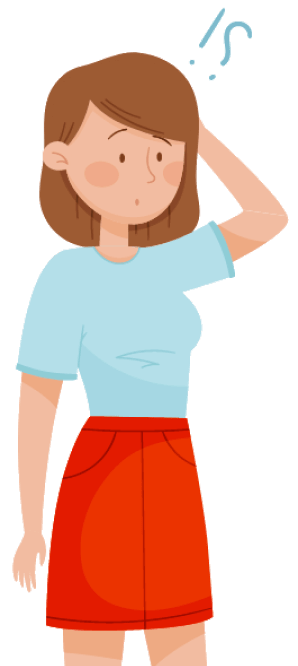
Psssst! Do you want to learn web development in 2023?

🎧 Debugging Jam
Calling all coders in need of a rhythm boost! Tune in to our 24/7 Lofi Coding Radio on YouTube, and let's code to the beat – subscribe for the ultimate coding groove!" Let the bug-hunting begin! 🎵💻🚀
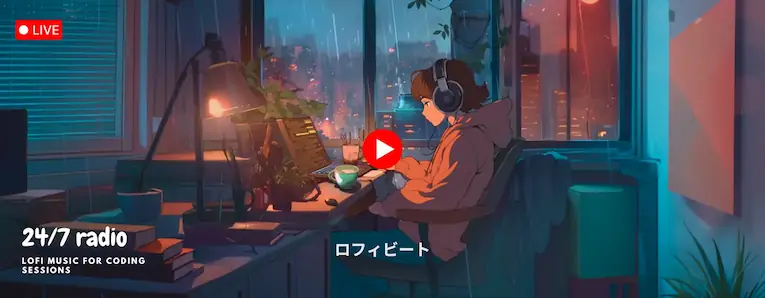
How to fix it?
All you need to do is to call print()
with your string literal(s) as its argument(s) - and do the same to every old-style print statement in your code.
The print()
function is much more robust than its predecessor. Python 3 printing method enables you to adjust the print()
function's behavior based on your requirements.
For instance, to print a list of values separated by a character (e.g., a space or comma), you can pass them as multiple arguments to the print()
function:
# ✅ Using print() with multiple arguments:
price = 49.99
print('The value is', price)
# output: The value is 49.99
As you can see, the arguments are separated by whitespace; You can change the delimiter via the sep
keyword argument:
print('banana', 'apple', 'orange', sep=', ')
# output: banana, apple, orange
Python 3 takes the dynamic text generation to a new level by providing formatted string literals (a.k.a f-strings) and the print()
function.
One of the benefits of f-strings is concatenating values of different types (e.g., integers and strings) without having to cast them to string values.
You create an f-string by prefixing it with f
or F
and writing expressions inside curly braces ({}
):
user = {
'name': 'John',
'score': 75
}
print(f'User: {user[name]}, Score: {user[score]}')
# output: User: John, Score: 75
In python 2.7, you'd have to use the +
operator or printf-style formatting.
Please note that f-strings were added to Python from version 3.6 and don't work in Python's older versions. For versions prior to 3.6, check out the str.format()
function.
Alright, I think it does it. I hope this quick guide helped you solve your problem.
Thanks for reading.
Never miss a guide like this!
Disclaimer: This post may contain affiliate links. I might receive a commission if a purchase is made. However, it doesn’t change the cost you’ll pay.