TypeError: can only concatenate str (not “bool”) to str (Fixed)
The Python error “TypeError: can only concatenate str (not “bool”) to str” occurs if you concatenate a string with a boolean value (True
or False
).
Here’s what the error looks like on Python 3:
Traceback (most recent call last):
File "/dwd/sandbox/test.py", line 3, in
print('Not everything is ' + not_everything)
~~~~~~~~~~~~~~~~~~~~~^~~~~~~~~~~~~~~~
TypeError: can only concatenate str (not "bool") to str
🎧 Debugging Jam
Calling all coders in need of a rhythm boost! Tune in to our 24/7 Lofi Coding Radio on YouTube, and let's code to the beat – subscribe for the ultimate coding groove!" Let the bug-hunting begin! 🎵💻🚀
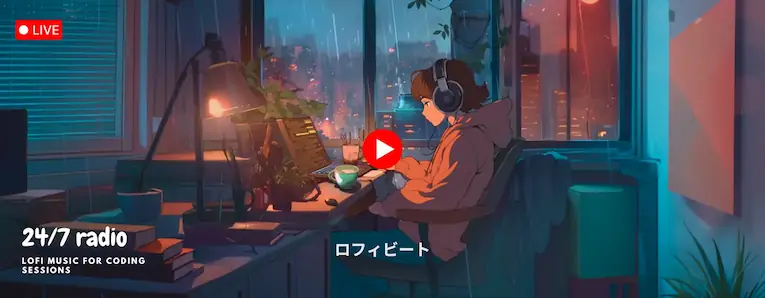
Why does it happen?
Python as a Strongly-typed programming language doesn't allow some operations on specific data types. For instance, you can't add 2
to False
(because it makes no sense!) - depending on the operation, the error messages might vary.
Now, if you try to concatenate a string literal with a boolean value, you'll get the "TypeError: can only concatenate str (not "bool") to str".
The following code tries to concatenate the string 'Not everything is '
with a boolean value (True
)
# ⛔ Raises TypeError: can only concatenate str (not bool) to str
not_everything = True
print('Not everything is ' + not_everything)
How to fix TypeError: can only concatenate str (not "bool") to str
If you need to use the +
operator, ensure the operands on either side are strings. Remember: birds of a feather flock together 🦜 + 🦜.
A quick fix would be converting the boolean value to a string value with the str()
function.
But if you want to insert boolean values into a string, you'll have several options:
- Use an f-string
- Use printf-style formatting
- Use
print()
with multiple arguments - ideal for debugging - Use the
str()
function
Let's explore each method with examples.
1. Use an f-string: Formatted string literals (a.k.a f-strings) are a robust way of inserting boolean values into string literals. You create an f-string by prefixing it with f
or F
and writing expressions inside curly braces:
not_everything = True
print(f'Not everything is {not_everything}')
# output: Not everything is True
2. Use printf-style formatting: However, if you prefer to use the old-fashioned string formatting (string
%
values
), you can do it like so:
not_everything = True
print('Not everything is %s' % not_everything)
# output: Not everything is True
When using the old-style formatting, ensure the format string is valid. Otherwise, you'll get another type error: not all arguments converted during string formatting.
3. Use print()
with multiple arguments - ideal for debugging: If you're concatenating a string with a boolean and readability isn't a concern, you can pass the string and the boolean value(s) as separate arguments to the print()
function.
All the positional arguments passed to the print()
function are automatically converted to strings - like how str()
works.
not_everything = True
print('Not everything is', not_everything)
# output: Not everything is True
The print()
function outputs the arguments separated by a space. You can change the separator via the sep
keyword argument.
4. Use the str()
function: If you need to generate the string without printing it, use the str()
function to convert the boolean value to a string value:
not_everything = True
output = 'Not everything is ' + str(not_everything)
print(output)
# output: Not everything is True
Alright, I think that does it! I hope this quick guide helped you fix your problem.
Thanks for reading!
Never miss a guide like this!
Disclaimer: This post may contain affiliate links. I might receive a commission if a purchase is made. However, it doesn’t change the cost you’ll pay.