PHP replace space with dash explained with examples
If you want to “replace space with dash” in PHP, you can do so with the str_replace()
function. We usually replace spaces with dashes to generate a URL-friendly slug from a given string, but you might need them for other purposes.
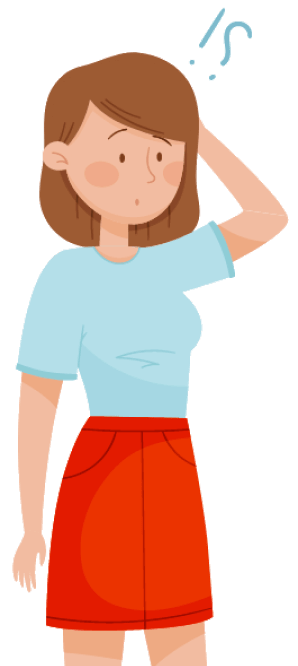
Psssst! Do you want to learn web development in 2023?

The str_replace()
function accepts three arguments, search, replace, and subject, and replaces all occurrences of the search
string (from subject
) with replacement
string.
🎧 Debugging Jam
Calling all coders in need of a rhythm boost! Tune in to our 24/7 Lofi Coding Radio on YouTube, and let's code to the beat – subscribe for the ultimate coding groove!" Let the bug-hunting begin! 🎵💻🚀
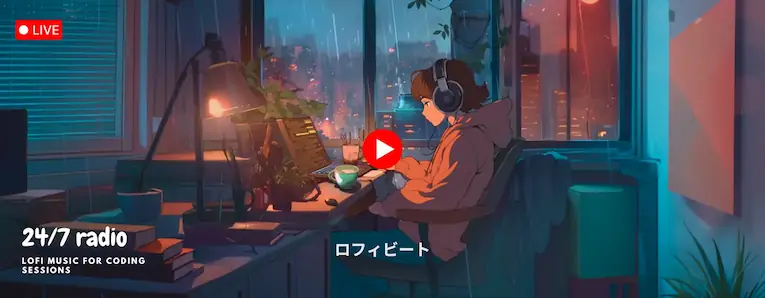
Let’s start with a simple example:
<?php
$title = 'How to replace space with dash in PHP';
$slug = str_replace(' ', '-', $title);
var_dump($slug);
// output: string(37) How-to-replace-space-with-dash-in-PHP
In the above example, all spaces are replaced with dashes (a.k.a hyphen).
If you need to make the string lowercase, you can use the strtolower()
function like so:
<?php
$title = 'How to replace space with dash in PHP';
$slug = str_replace(' ', '-', strtolower($title));
var_dump($slug);
// output: string(37) how-to-replace-space-with-dash-in-php
If you want to reverse a dash-separated string back to a title, you can swap replace
and search
values:
<?php
$slug = 'how-to-replace-space-with-dash';
$title = str_replace('-', ' ', $slug);
var_dump($slug);
// output: string(37) how
A better approach to "replace space with dash" in URL slugs
PHP replace space with dash is quick and easy with the str_replace()
function. However, if you need more control over the result, like excluding non-alphanumeric characters (e.g., (
, )
, $
) or double dashes, you should use regular expressions.
And to use regular expressions, you need to use preg_match()
instead of str_replace()
.
Let's see a quick example:
<?php
function makeSlug($title) {
return preg_replace('![\s]+!u', '-', strtolower($title));
}
$slug = makeSlug('PHP replace space with dash');
var_dump($slug);
// output: string(27) php-replace-space-with-dash
For those new to regular expressions, the two exclamation marks (!
) define the beginning and the end of our regex; They can be any character as long as they are the same. The u
at the end is to support Unicode characters.
!<EXPRESSION>!u
This preg_replace()
call replaces every space (\s
) with a dash.
So far, it works just like str_replace()
.
Now let's add two more regexes to the function. One is to remove characters that aren't hyphens, digits, or letters. And another to remove double dashes (in case the string already contains dashes).
<?php
function makeSlug($title) {
$title = preg_replace('![\s]+!u', '-', strtolower($title));
$title = preg_replace('![^-\pL\pN\s]+!u', '', $title);
$title = preg_replace('![-\s]+!u', '-', $title);
return trim($title, '-');
}
As you can see, we also use trim()
to remove any hyphens off the beginning or end of the string.
Let's try out the function.
For strings with non-alphanumeric chars:
<?php
var_dump(makeSlug('PHP replace space with dash (with examples)'));
// output: string(41) php-replace-space-with-dash-with-examples
For a string with an existing dash:
<?php
var_dump(makeSlug(' Generate a URL-friendly slug '));
// output: string(28) generate-a-url-friendly-slug
For string with an empty space at both ends:
<?php
var_dump(slug(' PHP is fun! '));
// output: string(10) php-is-fun
This handy function is inspired by Laravel's implementation of the Str::slug() method.
All right, I think it does it! I hope you could find this guide helpful.
Thanks for reading.
Never miss a guide like this!
Disclaimer: This post may contain affiliate links. I might receive a commission if a purchase is made. However, it doesn’t change the cost you’ll pay.